__
/ /________ _________ _____ _____ _
/ / ___/ __ \/ ___/ __ `/ __ `/ __ `/
/ (__ ) /_/ (__ ) /_/ / /_/ / /_/ /
/_/____/ .___/____/\__,_/\__, /\__,_/
/_/ /____/
⚡ designed for convenience and efficiency ⚡
A light-weight lsp plugin based on neovim's built-in lsp with a highly performant UI.
you can use some plugins management like lazy.nvim
, packaer.nvim
to install lspsaga
and lazyload by the plugin management keyword.
cmd
lazylod bylspsaga
commandft
lazy.nvim and packer both provide lazyload by filetype. then you can load the lspsaga according the filetypes which you write and use lsp.event
lazyload by event likeBufRead,BufReadPost
make sure your lsp plugin also loaded.dependices
for lazy.nvim you can set thelspsaga
intonvim-lspconfig
or other lsp plugindependices
keyword.after
for packer you can use after keyword.
require('lazy').setup({
'glepnir/lspsaga.nvim',
event = 'BufRead',
config = function()
require('lspsaga').setup({})
end
},opt)
use({
"glepnir/lspsaga.nvim",
branch = "main",
config = function()
require('lspsaga').setup({})
end,
})
require('lazy').setup({
'glepnir/lspsaga.nvim',
event = 'BufRead',
config = function()
require('lspsaga').setup({})
end
})
local keymap = vim.keymap.set
-- Lsp finder find the symbol definition implement reference
-- if there is no implement it will hide
-- when you use action in finder like open vsplit then you can
-- use <C-t> to jump back
keymap("n", "gh", "<cmd>Lspsaga lsp_finder<CR>")
-- Code action
keymap({"n","v"}, "<leader>ca", "<cmd>Lspsaga code_action<CR>")
-- Rename
keymap("n", "gr", "<cmd>Lspsaga rename<CR>")
-- Peek Definition
-- you can edit the definition file in this flaotwindow
-- also support open/vsplit/etc operation check definition_action_keys
-- support tagstack C-t jump back
keymap("n", "gd", "<cmd>Lspsaga peek_definition<CR>")
-- Go to Definition
keymap("n","gd", "<cmd>Lspsaga goto_definition<CR>")
-- Show line diagnostics you can pass arugment ++unfocus to make
-- show_line_diagnsotic float window unfocus
keymap("n", "<leader>sl", "<cmd>Lspsaga show_line_diagnostics<CR>")
-- Show cursor diagnostic
-- also like show_line_diagnostics support pass ++unfocus
keymap("n", "<leader>sc", "<cmd>Lspsaga show_cursor_diagnostics<CR>")
-- Show buffer diagnostic
keymap("n", "<leader>sb", "<cmd>Lspsaga show_buf_diagnostics<CR>")
-- Diagnsotic jump can use `<c-o>` to jump back
keymap("n", "[e", "<cmd>Lspsaga diagnostic_jump_prev<CR>")
keymap("n", "]e", "<cmd>Lspsaga diagnostic_jump_next<CR>")
-- Diagnostic jump with filter like Only jump to error
keymap("n", "[E", function()
require("lspsaga.diagnostic").goto_prev({ severity = vim.diagnostic.severity.ERROR })
end)
keymap("n", "]E", function()
require("lspsaga.diagnostic").goto_next({ severity = vim.diagnostic.severity.ERROR })
end)
-- Toglle Outline
keymap("n","<leader>o", "<cmd>Lspsaga outline<CR>")
-- Hover Doc
keymap("n", "K", "<cmd>Lspsaga hover_doc<CR>")
-- Callhierarchy
keymap("n", "<Leader>ci", "<cmd>Lspsaga incoming_calls<CR>")
keymap("n", "<Leader>co", "<cmd>Lspsaga outgoing_calls<CR>")
-- Float terminal
keymap({"n", "t"} "<A-d>", "<cmd>Lspsaga term_toggle<CR>")
Notice that title in float window must need neovim version >= 0.9
preview = {
lines_above = 0,
lines_below = 10,
},
scroll_preview = {
scroll_down = '<C-f>',
scroll_up = '<C-b>',
},
request_timeout = 2000,
Finder
to show the defintion,reference,implement(only show when current word is interface or some type)
default finder options
finder = {
edit = { 'o', '<CR>' },
vsplit = 's',
split = 'i',
tabe = 't',
quit = { 'q', '<ESC>' },
},
there has two commands Lspsaga peek_defintion
and Lspsaga goto_defintion
, the peek_defitnion
work like vscode that show the target file in a floatwindow you can edit as normalize.
options with default value
definition = {
edit = '<C-c>o',
vsplit = '<C-c>v',
split = '<C-c>i',
tabe = '<C-c>t',
quit = 'q',
close = '<Esc>',
}
peek_definition show case
the step in this gif show case
gd
runlspsaga peek_definition
.- do some comment edit then
:w
to save. <C-c> o
jump to this file.- lspsaga will show becacon highlight after jump .
jump to definition and show beacon
options with default value
code_action = {
num_shortcut = true,
keys = {
quit = 'q',
exec = '<CR>',
},
},
num_shortcut
it'strue
by default then you can use number to fast run action
code_action show case
ga
runLspsaga code_action
j
to move and show the code action preview<Cr>
to run a action
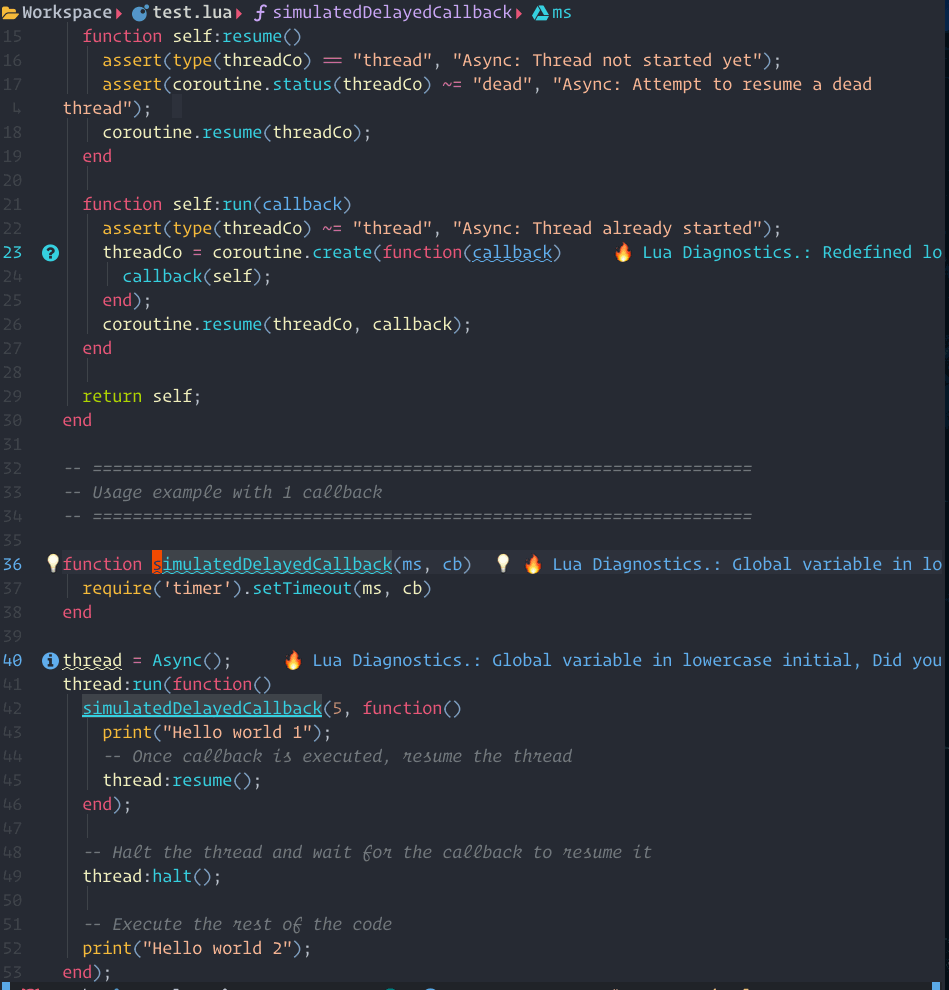
when there has code action it will show a lightbulb, default options.
lightbulb = {
enable = true,
enable_in_insert = true,
sign = true,
sign_priority = 40,
virtual_text = true,
},
lspsaga use treesitter markdown parser to render hover. so you must install markdown parser.
you can press shotcut of Lspsaga hover_doc
twice jump into the hover window. in my case is K
.
hover_doc show case
K
to runLspsaga hover_doc
.- press
K
again jump into the hover window. q
to quit.
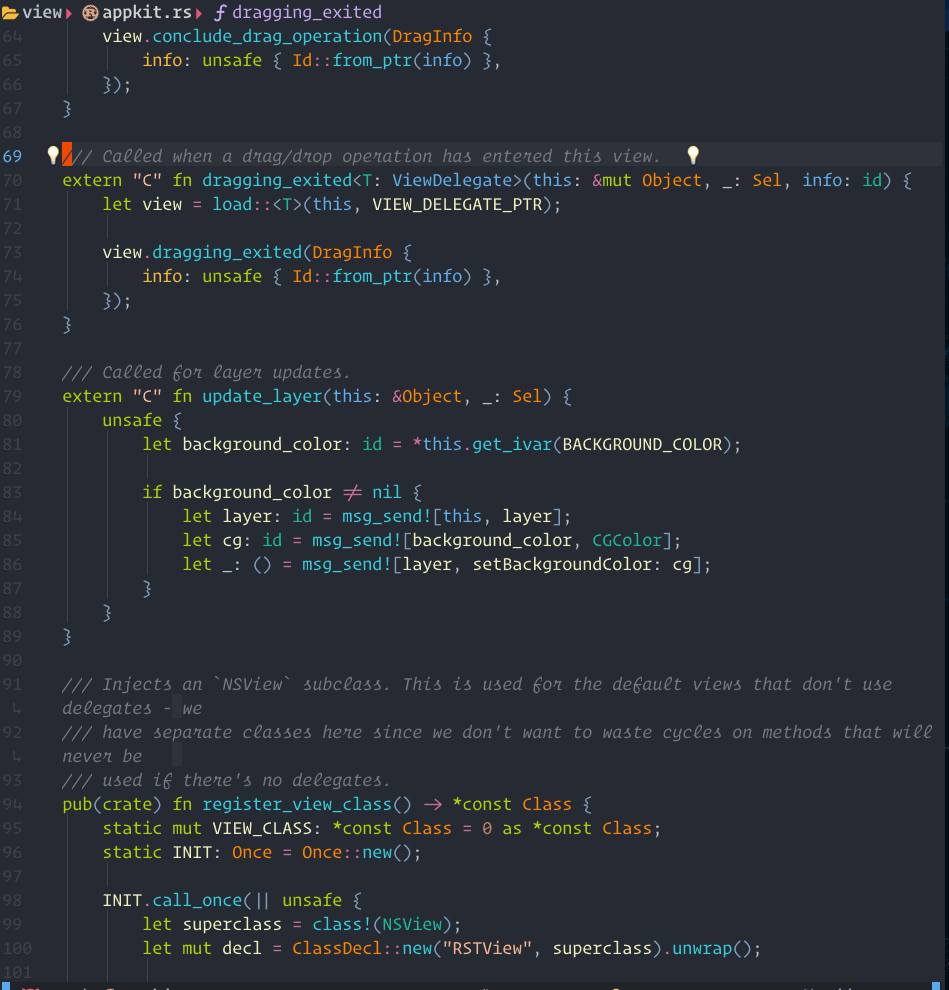
jump to next diagnsotic position then show beacon and show the codeaction. default options
diagnostic = {
twice_into = false,
show_code_action = true,
show_source = true,
keys = {
exec_action = 'o',
quit = 'q',
},
},
if twice_into
set to true
, press twice the diagnostic jump shortcut it will jump into the floatwindow. other way is use wincmd
to jump into use <C-w>w
.
also you can use a filter in diagnostic jump by using lspsaga function. function params is a table
same as :h vim.diagnsotic.get_next
-- this is mean only jump to error position
-- or goto_next
require("lspsaga.diagnostic").goto_prev({ severity = vim.diagnostic.severity.ERROR })
diagnostic jump show case
[e
to jump next diangostic position.<C-w>w
jump into the float window.j
to move.o
to execuate an action.
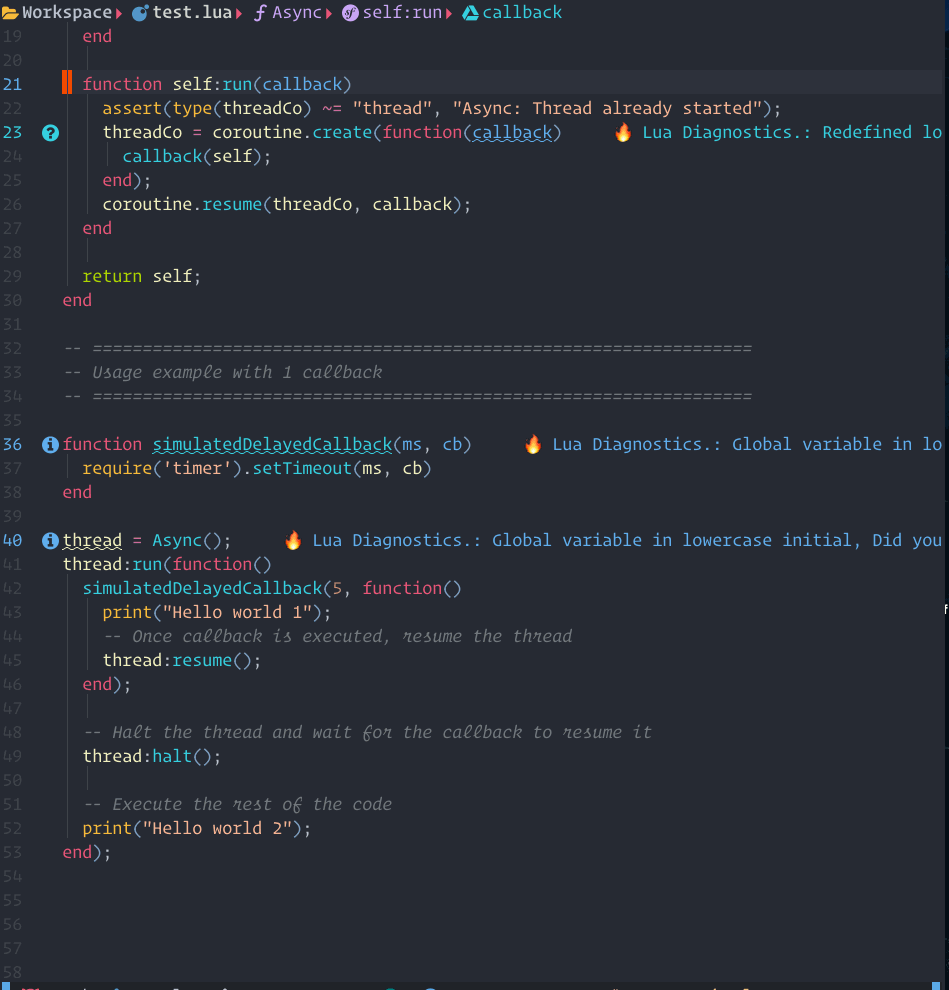
show_line_diagnsotic
, show_buf_diagnostics
,show_cursor_diagnostics
lsp rename with select. default options
rename = {
quit = '<C-c>',
exec = '<CR>',
in_select = true,
},
default options
outline = {
win_position = 'right',
win_with = '',
win_width = 30,
show_detail = true,
auto_preview = true,
auto_refresh = true,
auto_close = true,
custom_sort = nil,
keys = {
jump = 'o',
expand_collaspe = 'u',
quit = 'q',
},
},
run lsp callhierarchy incoming_calls. default options
callhierarchy = {
show_detail = false,
keys = {
edit = 'e',
vsplit = 's',
split = 'i',
tabe = 't',
jump = 'o',
quit = 'q',
expand_collaspe = 'u',
},
},
run lsp callhierarchy outgoing_calls
require your neovim version >= 0.8. options with default value
symbol_in_winbar = {
enable = true,
separator = ' ',
hide_keyword = true,
show_file = true,
folder_level = 2,
},
hide_keyword
default is true it will hide some keyword or tmp variable make symbols more cleanfolder_level
work withshow_file
lspsaga provide an api that you can use in your custom winbar or statusline.
vim.wo.winbar/ vim.wo.stl = require('lspsaga.symbolwinbar'):get_winbar()
simple floaterm
default ui options
ui = {
-- currently only round theme
theme = 'round',
-- border type can be single,double,rounded,solid,shadow.
border = 'solid',
winblend = 0,
expand = '',
collaspe = '',
preview = ' ',
code_action = '💡',
diagnostic = '🐞',
incoming = ' ',
outgoing = ' ',
colors = {
--float window normal bakcground color
normal_bg = '#1d1536',
--title background color
title_bg = '#afd700',
red = '#e95678',
magenta = '#b33076',
orange = '#FF8700',
yellow = '#f7bb3b',
green = '#afd700',
cyan = '#36d0e0',
blue = '#61afef',
purple = '#CBA6F7',
white = '#d1d4cf',
black = '#1c1c19',
},
kind = {},
},
you can change the default colors or only change some highlight groups which you want change. find all highlight groups in highlight.lua
kind
field is a table that key is kind name, value is icon, all kind defined in lspkind.lua
If you'd like to support my work financially, buy me a drink through paypal.
Licensed under the MIT license.