forked from astiopin/webgl_fonts
-
Notifications
You must be signed in to change notification settings - Fork 1
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
12 changed files
with
152 additions
and
90 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,2 +1,3 @@ | ||
node_modules | ||
dist | ||
dist | ||
.vercel |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,19 +1,63 @@ | ||
# WebGL Font Rendering | ||
|
||
Demonstration of a font rendering on the GPU with glyph hinting and subpixel antialiasing. | ||
Crisp font rendering on the GPU with glyph hinting and subpixel antialiasing. No external dependencies, very small (3.8kb gzipped). | ||
|
||
## Usage | ||
|
||
```bash | ||
npm install webgl-fonts | ||
``` | ||
|
||
Place your fonts in the `public/fonts` or `static/fonts` directory depending on your setup. Then use `loadFont` function to load the font and `createRenderer` to create a renderer. | ||
|
||
```javascript | ||
import { createRenderer, loadFont } from 'webgl-fonts'; | ||
|
||
// create a WebGL2 context | ||
const canvas = document.getElementById('canvas'); | ||
const gl = canvas.getContext('webgl2', { | ||
premultipliedAlpha: false, | ||
alpha: false, | ||
}); | ||
|
||
// thiis will load json and png files from | ||
// public/fonts/roboto.json and public/fonts/roboto.png | ||
const font = await loadFont(gl, 'roboto'); | ||
const renderer = createRenderer(gl); | ||
|
||
// render loop | ||
function loop() { | ||
renderer.render({ | ||
font, | ||
font_size: 32, | ||
text: 'Hello, world!', | ||
x: 0, | ||
y: 0, | ||
font_hinting: true, | ||
subpixel: true, | ||
font_color: [1, 1, 1, 1], | ||
bg_color: [0, 0, 0, 1], | ||
}); | ||
requestAnimationFrame(loop); | ||
} | ||
loop(); | ||
|
||
``` | ||
|
||
## Demo | ||
|
||
The demo uses [signed distance field method](http://www.valvesoftware.com/publications/2007/SIGGRAPH2007_AlphaTestedMagnification.pdf) for glyph rendering. | ||
|
||
[Click here to see the demo](http://astiopin.github.io/webgl_fonts) (requires WebGL). | ||
[Click here to see the demo](https://webgl-fonts.vercel.app/) (requires WebGL). | ||
|
||
Font atlas generation tool is [here](https://github.com/astiopin/sdf_atlas). | ||
|
||
## Hinting | ||
|
||
The idea is pretty simple. First we're placing the text baseline exactly at the pixel boundary. Next we're using two different methods to place the glyphs. Lowcase characters are scaled in a such way that the [x-height](https://en.wikipedia.org/wiki/X-height) spans a whole number of pixels. All other characters are scaled to fit the [cap height](https://en.wikipedia.org/wiki/Cap_height) to the pixel boundary. | ||
|
||
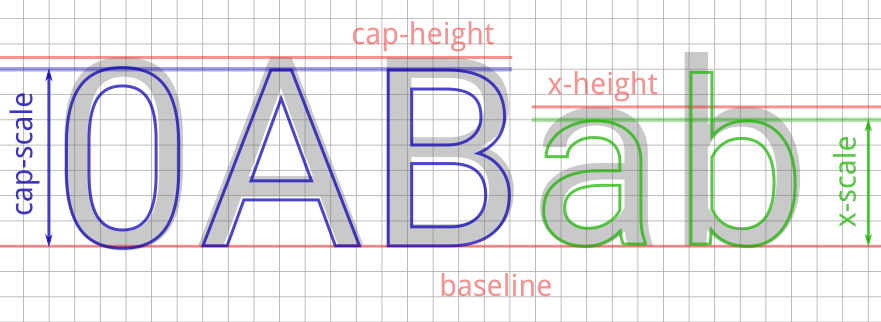 | ||
 | ||
|
||
At the rasterisation stage we're modifying the antialiazing routine so that the antialiazed edge distance depends on a stroke direction, which makes horizontal strokes appear sharper than the vertical ones. | ||
|
||
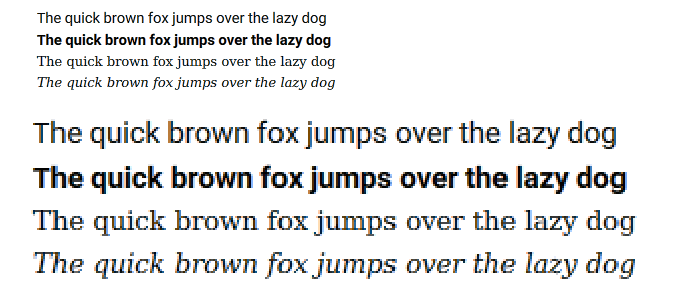 | ||
 |
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -9,6 +9,9 @@ | |
"build": "vite build" | ||
}, | ||
"author": "Anton Stepin <[email protected]>", | ||
"contributors": [ | ||
"Máté Homolya <[email protected]>" | ||
], | ||
"license": "See LICENSE in LICENSE", | ||
"devDependencies": { | ||
"vite": "^4.3.9", | ||
|
File renamed without changes.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.