- Tiny with 0 dependency and simple (715B gzip)
- Persist state by default (
sessionStorage
orlocalStorage
) - Build with React Hooks
$ npm install little-state-machine
This is a Provider Component to wrapper around your entire app in order to create context.
<StateMachineProvider>
<App />
</StateMachineProvider>
Function to initialize the global store, invoked at your app root (where <StateMachineProvider />
lives).
function log(store) {
console.log(store);
return store;
}
createStore(
{
yourDetail: { firstName: '', lastName: '' } // it's an object of your state
},
{
name?: string; // rename the store
middleWares?: [ log ]; // function to invoke each action
storageType?: Storage; // session/local storage (default to session)
persist?: 'action' // onAction is default if not provided
// when 'none' is used then state is not persisted
// when 'action' is used then state is saved to the storage after store action is completed
// when 'beforeUnload' is used then state is saved to storage before page unloa
},
);
This hook function will return action/actions and state of the app.
const { actions, state, getState } = useStateMachine<T>({
updateYourDetail,
});
Check out the Demo.
import React from 'react';
import {
StateMachineProvider,
createStore,
useStateMachine,
} from 'little-state-machine';
createStore({
yourDetail: { name: '' },
});
function updateName(state, payload) {
return {
...state,
yourDetail: {
...state.yourDetail,
...payload,
},
};
}
function YourComponent() {
const { actions, state } = useStateMachine({ updateName });
return (
<div onClick={() => actions.updateName({ name: 'bill' })}>
{state.yourDetail.name}
</div>
);
}
const App = () => (
<StateMachineProvider>
<YourComponent />
</StateMachineProvider>
);
You can create a global.d.ts
file to declare your GlobalState's type.
Checkout the example.
import 'little-state-machine';
declare module 'little-state-machine' {
interface GlobalState {
yourDetail: {
name: string;
};
}
}
Quick video tutorial on little state machine.
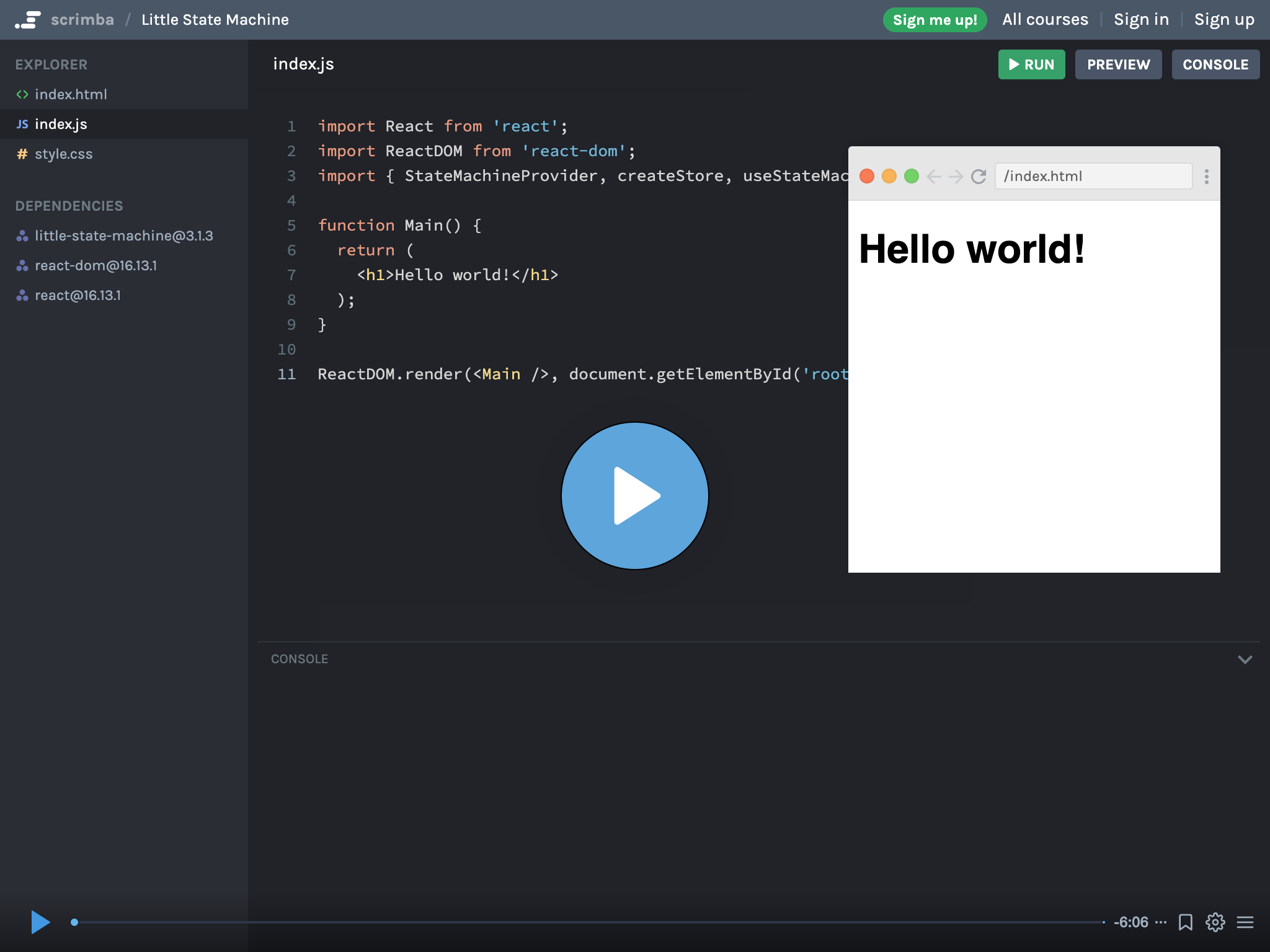
DevTool component to track your state change and action.
import { DevTool } from 'little-state-machine-devtools';
<StateMachineProvider>
<DevTool />
</StateMachineProvider>;
We also make BEEKAI. Build the next-generation forms with modern technology and best in class user experience and accessibility.
Thanks go to these wonderful people: