|
1 | 1 | # jquery.dad.js
|
| 2 | + |
2 | 3 | DAD: A simple and awesome Drag And Drop plugin!
|
3 | 4 |
|
4 |
| -## 1.Installation |
5 |
| -Insert the basic css file: |
6 |
| -``` |
7 |
| -<link rel="stylesheet" href="jquery.dad.css"> |
8 |
| -```` |
| 5 | +## Table of contents |
| 6 | + |
| 7 | +- [Installation](#installation) |
| 8 | +- [Usage](#usage) |
| 9 | +- [Options](#options) |
| 10 | + - [active](#active) |
| 11 | + - [draggable](#draggable) |
| 12 | + - [exchangeable](#exchangeable) |
| 13 | + - [transition](#transition) |
| 14 | + - [placeholderTarget](#placeholdertarget) |
| 15 | + - [placeholderTemplate](#placeholdertemplate) |
| 16 | +- [Methods](#methods) |
| 17 | + - [activate](#activate) |
| 18 | + - [deactivate](#deactivate) |
| 19 | +- [Events](#events) |
| 20 | + - [dadDropStart](#daddropstart) |
| 21 | + - [dadDropStart](#daddropstart-1) |
| 22 | +- [Demos](#demos) |
| 23 | + |
| 24 | +## Installation |
| 25 | + |
9 | 26 | And the dad plugin file after jquery.
|
| 27 | + |
10 | 28 | ```
|
11 | 29 | <script src='jquery.min.js'></script>
|
12 |
| -<script src='jquery.dad.js'></script> |
| 30 | +<script src='jquery.dad.min.js'></script> |
13 | 31 | ```
|
14 |
| -## 2.Usage |
| 32 | + |
| 33 | +## Usage |
| 34 | + |
15 | 35 | Create a group of DOM elements that can be resorted via drag and drop inside the parent container 'demo'.
|
| 36 | + |
16 | 37 | ```
|
| 38 | +
|
17 | 39 | <div class="demo">
|
18 | 40 | <div>...</div>
|
19 | 41 | <div>...</div>
|
20 | 42 | <div>...</div>
|
21 | 43 | <div>...</div>
|
22 | 44 | </div>
|
| 45 | +
|
23 | 46 | ```
|
| 47 | + |
24 | 48 | and just call it:
|
| 49 | + |
25 | 50 | ```
|
26 |
| -$(function(){ |
27 |
| - $('.demo').dad(options); |
28 |
| -}) |
| 51 | +
|
| 52 | +$('.my-container').dad(); |
| 53 | +
|
29 | 54 | ```
|
30 |
| -## 3.Options |
| 55 | + |
| 56 | +## Options |
| 57 | + |
31 | 58 | You can call options width a JSON object.
|
32 |
| -* target: '.selector'; |
33 |
| -* draggable: '.selector' from the target child div. |
34 |
| -* callback: function(e){} where e is the jquery object for the dropped element |
35 |
| -* placeholder: string with the placeholder text on draggable area |
36 |
| -
|
37 |
| -## 4.Functions |
38 |
| -* n.addDropzone(selector,function(e){}); |
39 |
| -Sample: |
40 |
| -``` |
41 |
| -var n=$('.demo').dad(); |
42 |
| -n.addDropzone('.dropzone',function(e){ |
43 |
| - console.log(e); //e is the jquery object for the dropped element |
| 59 | + |
| 60 | +### active |
| 61 | + |
| 62 | +**Type:** `boolean` |
| 63 | +**Default value:** `false` |
| 64 | + |
| 65 | +**Description:** |
| 66 | + |
| 67 | +Setting it to false prevents the containers to start dragging. You can check if a container is enabled or disabled by the attribute `dada-dat-active` or using the instance like: |
| 68 | + |
| 69 | +``` |
| 70 | +
|
| 71 | +var instance = $(".my-container").dad({ active: false }); |
| 72 | +console.log(instance.active); // prints false |
| 73 | +
|
| 74 | +``` |
| 75 | + |
| 76 | +### draggable |
| 77 | + |
| 78 | +**Type:** `string`_(selector)_ | `false` |
| 79 | +**Default value:** `false` |
| 80 | + |
| 81 | +**Description:** |
| 82 | + |
| 83 | +Passing a selector restricts the drag to start only when the element from the given selector is clicked within the child element. |
| 84 | +If `false` fallsback to the target child element. |
| 85 | + |
| 86 | +``` |
| 87 | +
|
| 88 | +// the drag will only start when mouse is hovering .my-drag-selector |
| 89 | +var instance = $(".my-container").dad({ |
| 90 | + draggable: ".my-drag-selector" |
| 91 | +}); |
| 92 | +
|
| 93 | +``` |
| 94 | + |
| 95 | +### exchangeable |
| 96 | + |
| 97 | +**Type:** `boolean` |
| 98 | +**Default value:** `true` |
| 99 | + |
| 100 | +**Description:** |
| 101 | + |
| 102 | +Allows containers of the same type _(called with the same `.dad` call)_ to exchange children. |
| 103 | + |
| 104 | +### transition |
| 105 | + |
| 106 | +**Type:** `number` |
| 107 | +**Default value:** `200` |
| 108 | + |
| 109 | +**Description:** |
| 110 | + |
| 111 | +Transition time in ms of the drag animation |
| 112 | + |
| 113 | +### placeholderTarget |
| 114 | + |
| 115 | +**Type:** `string`_(selector)_ | `false` |
| 116 | +**Default value:** `false` |
| 117 | + |
| 118 | +**Description:** |
| 119 | + |
| 120 | +The target element within the current child to be covered by the placeholder. |
| 121 | + |
| 122 | +### placeholderTemplate |
| 123 | + |
| 124 | +**Type:** `string`_(selecor)_ |
| 125 | +**Default value:** `<div />` |
| 126 | + |
| 127 | +**Description:** |
| 128 | + |
| 129 | +Enable the full customization of the placeholder by passing a HTML string. E.g. |
| 130 | + |
| 131 | +``` |
| 132 | +
|
| 133 | +$(".my-container").dad({ |
| 134 | + placeholderTemplate: "<div style=\"border: 1px dashed black\">i'm a placeholder</div>" |
44 | 135 | })
|
| 136 | +
|
| 137 | +``` |
| 138 | + |
| 139 | +## Methods |
| 140 | + |
| 141 | +### activate |
| 142 | + |
| 143 | +Set the container's `active` state to `true` |
| 144 | + |
45 | 145 | ```
|
46 |
| -* n.activate() |
| 146 | +
|
| 147 | +var instance = $('.my-container').dad(); |
| 148 | +instance.activate(); |
| 149 | +
|
| 150 | +``` |
| 151 | + |
| 152 | +### deactivate |
| 153 | + |
| 154 | +Set the container's `active` state to `false` |
| 155 | + |
47 | 156 | ```
|
48 |
| -var n=$('.demo').dad(); |
49 |
| -n.activate(); |
| 157 | +
|
| 158 | +var instance = $('.my-container').dad(); |
| 159 | +instance.deactivate(); |
| 160 | +
|
50 | 161 | ```
|
51 |
| -* n.deactivate() |
| 162 | + |
| 163 | +## Events |
| 164 | + |
| 165 | +### dadDropStart |
| 166 | + |
| 167 | +This event is triggered imediatelly after the user drops the element. The callback sends the dropped element (as DOM node) as the second argument. |
| 168 | + |
52 | 169 | ```
|
53 |
| -var n=$('.demo').dad(); |
54 |
| -n.deactivate(); |
| 170 | +
|
| 171 | +$(".my-container").on("dadDropStart", function (e, droppedElement) { |
| 172 | + // do your thing here |
| 173 | +}) |
| 174 | +
|
55 | 175 | ```
|
56 | 176 |
|
| 177 | +### dadDropStart |
57 | 178 |
|
58 |
| -For more info visit the [plugin website](http://konsole.studio/dad) |
| 179 | +This event is triggered when the dropping animation ends. The callback sends the dropped element (as DOM node) as the second argument as well. |
59 | 180 |
|
| 181 | +``` |
| 182 | +
|
| 183 | +$(".my-container").on("dadDropEnd", function (e, droppedElement) { |
| 184 | + // do your thing here |
| 185 | +}) |
| 186 | +
|
| 187 | +``` |
60 | 188 |
|
61 |
| -[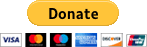](https://www.paypal.com/cgi-bin/webscr?cmd=_donations&business=MRH3WC7BR5WEE&lc=US&item_name=jquery%2edad%2ejs¤cy_code=USD&bn=PP%2dDonationsBF%3abtn_donateCC_LG%2egif%3aNonHosted) |
| 189 | +## Demos |
62 | 190 |
|
| 191 | +Check the demos at [plugin website](http://konsole.studio/dad) |
0 commit comments