You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Hi all.
I tried creating a RoundRaisedButton with text, and then use it as a MapMarker and MapMarkerPopup itself instead of using an icon with source.
Creating the Button worked fine as shown below:
`from kivy.lang import Builder
from kivy.uix.behaviors import ButtonBehavior
from kivymd.uix.boxlayout import MDBoxLayout
from kivymd.app import MDApp
from kivymd.uix.behaviors import (
CircularRippleBehavior,
FakeCircularElevationBehavior,
)
class CircularElevationButton(
FakeCircularElevationBehavior,
CircularRippleBehavior,
ButtonBehavior,
MDBoxLayout,
):
pass
class Example(MDApp):
def build(self):
return Builder.load_string(KV)
Example().run()`
But after adding it as a child widget under MapView to replace the regular MapMarkerPopup, the text became redundant. I don't know if i am not doing it right, or otherwise.
Below is a code of how I have tried implementing it with MapView, and any helpful pointer towards a better solution will be very much appreciated.
`from kivy.lang import Builder
from kivy.uix.behaviors import ButtonBehavior
from kivy.garden.mapview import MapMarkerPopup
from kivymd.uix.boxlayout import MDBoxLayout
from kivymd.app import MDApp
from kivymd.uix.behaviors import (
CircularRippleBehavior,
FakeCircularElevationBehavior,
)
Hi all.
I tried creating a RoundRaisedButton with text, and then use it as a MapMarker and MapMarkerPopup itself instead of using an icon with source.
Creating the Button worked fine as shown below:
`from kivy.lang import Builder
from kivy.uix.behaviors import ButtonBehavior
from kivymd.uix.boxlayout import MDBoxLayout
from kivymd.app import MDApp
from kivymd.uix.behaviors import (
CircularRippleBehavior,
FakeCircularElevationBehavior,
)
KV = '''
:
size_hint: None, None
size: "25dp", "25dp"
radius: self.size[0] / 2
md_bg_color: 1, 0, 0, 1
MDLabel:
text: "[b]DC[/b]"
markup: True
halign: "center"
valign: "center"
size: root.size
pos: root.pos
font_size: root.size[0] * .6
theme_text_color: "Custom"
text_color: [1] * 4
MDScreen:
'''
class CircularElevationButton(
FakeCircularElevationBehavior,
CircularRippleBehavior,
ButtonBehavior,
MDBoxLayout,
):
pass
class Example(MDApp):
def build(self):
return Builder.load_string(KV)
Example().run()`
But after adding it as a child widget under MapView to replace the regular MapMarkerPopup, the text became redundant. I don't know if i am not doing it right, or otherwise.
Below is a code of how I have tried implementing it with MapView, and any helpful pointer towards a better solution will be very much appreciated.
`from kivy.lang import Builder
from kivy.uix.behaviors import ButtonBehavior
from kivy.garden.mapview import MapMarkerPopup
from kivymd.uix.boxlayout import MDBoxLayout
from kivymd.app import MDApp
from kivymd.uix.behaviors import (
CircularRippleBehavior,
FakeCircularElevationBehavior,
)
KV = '''
:
size_hint: None, None
size: "25dp", "25dp"
radius: self.size[0] / 2
md_bg_color: 1, 0, 0, 1
MDLabel:
text: "[b]DC[/b]"
markup: True
halign: "center"
valign: "center"
size: root.size
pos: root.pos
font_size: root.size[0] * .6
theme_text_color: "Custom"
text_color: [1] * 4
MapView:
lat: 10
lon: 10
zoom: 5
CircularElevationButton:
lat: 12
lon: 12
elevation: 5
'''
class CircularElevationButton(
MapMarkerPopup,
FakeCircularElevationBehavior,
CircularRippleBehavior,
ButtonBehavior,
MDBoxLayout,
):
pass
class Example(MDApp):
def build(self):
return Builder.load_string(KV)
Example().run()`
Below is an image of what i am trying to replicate as a Marker
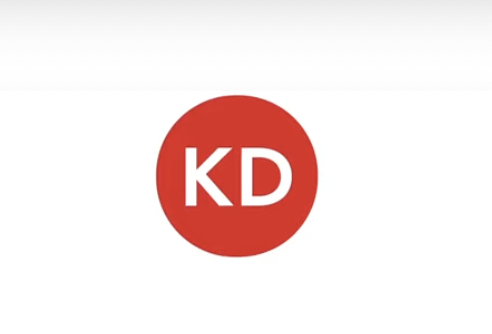
.
The text was updated successfully, but these errors were encountered: